JavaScript : Create a function that invokes the provided function with its arguments transformed
JavaScript fundamental (ES6 Syntax): Exercise-172 with Solution
Function with Transformed Arguments
Write a JavaScript program to create a function that invokes the provided function with its arguments transformed.
- Use Array.prototype.map() to apply transforms to args in combination with the spread operator (...) to pass the transformed arguments to fn.
Sample Solution:
JavaScript Code:
// Define a function 'overArgs' that takes a function 'fn' and an array of transformation functions 'transforms'
const overArgs = (fn, transforms) =>
// Return a new function that takes any number of arguments
(...args) =>
// Apply the original function 'fn' to the arguments after applying the corresponding transformation functions
fn(
// Map over the arguments and transform each one using the corresponding transformation function
...args.map((val, i) => transforms[i](val))
);
// Define a function 'square' that returns the square of its input
const square = n => n * n;
// Define a function 'double' that returns the double of its input
const double = n => n * 2;
// Define a new function 'fn' that takes two arguments and applies 'square' to the first argument and 'double' to the second
const fn = overArgs((x, y) => [x, y], [square, double]);
// Call 'fn' with two sample arguments and log the result
console.log(fn(9, 3)); // [81, 6]
console.log(fn(5, 2)); // [25, 4]
Output:
[81,6] [25,4]
Visual Presentation:
Flowchart:
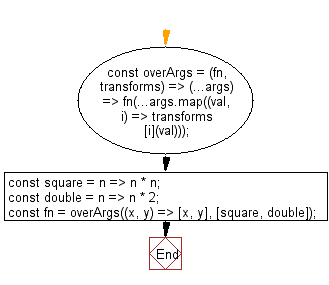
Live Demo:
See the Pen javascript-basic-exercise-172-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to parse an HTTP Cookie header string and return an object of all cookie name-value pairs.
Next: Write a JavaScript program to get the nth element of an given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics