JavaScript: Create a function that invokes fn with partials prepended to the arguments it receives
JavaScript fundamental (ES6 Syntax): Exercise-170 with Solution
Function with Prepended Partials
Write a JavaScript program to create a function that invokes fn with partials prepended to the arguments it receives.
- Use the spread operator (...) to prepend partials to the list of arguments of fn.
Sample Solution:
JavaScript Code:
// Define a function 'partial' that partially applies arguments to the left side of a function 'fn'
const partial = (fn, ...partials) => (...args) => fn(...partials, ...args);
// Define a function 'greet' that concatenates a 'greeting' string and a 'name' string with an exclamation mark
const greet = (greeting, name) => greeting + ' ' + name + '!';
// Partially apply the 'greeting' argument as 'Hello' to the 'greet' function using 'partial'
const greetHello = partial(greet, 'Hello');
// Call the partially applied function 'greetHello' with the 'name' argument as 'John'
console.log(greetHello('John'));
Output:
Hello John!
Flowchart:
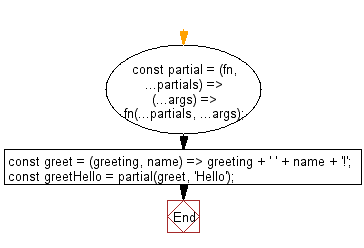
Live Demo:
See the Pen javascript-basic-exercise-170-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a new function which prepends fixed arguments to the arguments supplied during invocation.
- Write a JavaScript function that implements partial application by binding the first few parameters and deferring the rest.
- Write a JavaScript program that wraps a function to automatically add preset arguments at the beginning of its parameter list.
- Write a JavaScript function that shows how to prepend arguments to a function call and then passes the remaining arguments normally.
Go to:
PREV : Function with Appended Partials.
NEXT : Parse Cookie Header to Object.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.