JavaScript: Create a function that invokes fn with partials appended to the arguments it receives
JavaScript fundamental (ES6 Syntax): Exercise-169 with Solution
Function with Appended Partials
Write a JavaScript program to create a function that invokes fn with partials appended to the arguments it receives.
- Use the spread operator (...) to append partials to the list of arguments of fn.
Sample Solution:
JavaScript Code:
// Define a function 'partialRight' that partially applies arguments to the right side of a function 'fn'
const partialRight = (fn, ...partials) => (...args) => fn(...args, ...partials);
// Define a function 'greet' that concatenates a 'greeting' string and a 'name' string with an exclamation mark
const greet = (greeting, name) => greeting + ' ' + name + '!';
// Partially apply the 'name' argument as 'John' to the 'greet' function using 'partialRight'
const greetJohn = partialRight(greet, 'John');
// Call the partially applied function 'greetJohn' with the 'greeting' argument as 'Hello'
console.log(greetJohn('Hello'));
Output:
Hello John!
Flowchart:
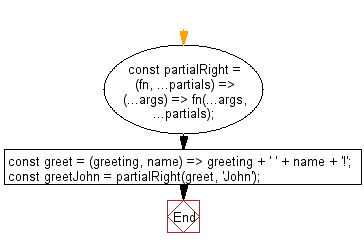
Live Demo:
See the Pen javascript-basic-exercise-169-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to group the elements into two arrays, depending on the provided function's truthiness for each element.
Next: Write a JavaScript program to create a function that invokes fn with partials prepended to the arguments it receives.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics