JavaScript: Group the elements into two arrays, depending on the provided function's truthiness for each element
JavaScript fundamental (ES6 Syntax): Exercise-168 with Solution
Write a JavaScript program to group the elements into two arrays, depending on the provided function's truthiness for each element.
- Use Array.prototype.reduce() to create an array of two arrays.
- Use Array.prototype.push() to add elements for which fn returns true to the first array and elements for which fn returns false to the second one.
Sample Solution:
JavaScript Code:
// Define a function 'partition' that partitions an array based on the result of a provided function 'fn'
const partition = (arr, fn) =>
// Use the reduce method to iterate over the array and partition its elements
arr.reduce(
// Callback function with accumulator 'acc', current value 'val', index 'i', and the original array 'arr'
(acc, val, i, arr) => {
// Push the current value 'val' into the appropriate partition array based on the result of 'fn(val, i, arr)'
acc[fn(val, i, arr) ? 0 : 1].push(val);
// Return the updated accumulator 'acc' for the next iteration
return acc;
},
// Initialize the accumulator as an array containing two empty arrays
[[], []]
);
// Define an array 'users' containing objects representing users with 'user', 'age', and 'active' properties
const users = [{ user: 'barney', age: 36, active: false }, { user: 'fred', age: 40, active: true }];
// Partition the 'users' array based on the 'active' property of each user object
partition(users, o => o.active);
// Log the original 'users' array
console.log(users);
Output:
[{"user":"barney","age":36,"active":false},{"user":"fred","age":40,"active":true}]
Flowchart:
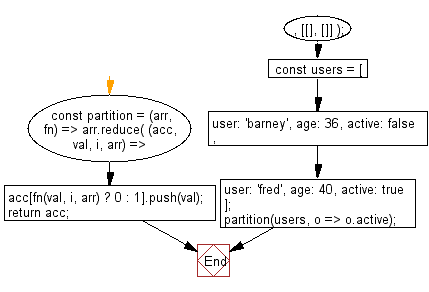
Live Demo:
See the Pen javascript-basic-exercise-168-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to calculate how many numbers in the given array are less or equal to the given value using the percentile formula.
Next: Write a JavaScript program to create a function that invokes fn with partials appended to the arguments it receives.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-168.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics