JavaScript: Calculate how many numbers in the given array are less or equal to the given value using the percentile formula
JavaScript fundamental (ES6 Syntax): Exercise-167 with Solution
Count Less-Than-or-Equal Numbers
Write a JavaScript program to calculate how many numbers in the given array are less than or equal to the given value. This is done using the percentile formula.
Use Array.prototype.reduce() to calculate how many numbers are below the value and how many are the same value and apply the percentile formula.
Sample Solution:
JavaScript Code:
// Define a function 'percentile' that calculates the percentile of a given number in an array
const percentile = (arr, num) => (arr.filter((item) => item <= num).length / arr.length) * 100;
// Calculate and log the percentile of the number 5 in the array [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
console.log(percentile([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 5)) // 50;
// Calculate and log the percentile of the number 10 in the array [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
console.log(percentile([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 10)) // 100;
// Calculate and log the percentile of the number 3 in the array [1, 2, 3, 4, 5, 6]
console.log(percentile([1, 2, 3, 4, 5, 6],3)) // 50;
// Calculate and log the percentile of the number 4 in the array [1, 2, 3, 4, 5, 6]
console.log(percentile([1, 2, 3, 4, 5, 6],4)) // 66.66;
Output:
50 100 50 66.66666666666666
Flowchart:
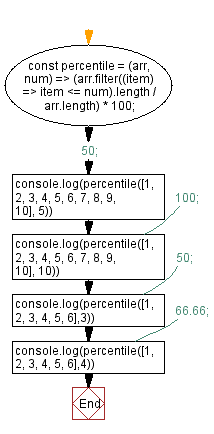
Live Demo:
See the Pen javascript-basic-exercise-167-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that counts how many numbers in an array are less than or equal to a given value using the percentile formula.
- Write a JavaScript function that iterates over an array and returns the count of elements meeting a threshold condition.
- Write a JavaScript program that computes the percentage of numbers below or equal to a target in a sorted array.
- Write a JavaScript function that calculates a cumulative frequency for numbers up to a specified limit in an array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform left-to-right function composition for asynchronous functions.
Next: Write a JavaScript program to group the elements into two arrays, depending on the provided function's truthiness for each element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.