JavaScript: Return the singular or plural form of the word based on the input number
JavaScript fundamental (ES6 Syntax): Exercise-164 with Solution
Write a JavaScript program that returns the singular or plural form of the word based on the input number.
If the first argument is an object, it will use a closure by returning a function that can auto-pluralize words that don't simply end in s if the supplied dictionary contains the word.
- Use a closure to define a function that pluralizes the given word based on the value of num.
- If num is either -1 or 1, return the singular form of the word.
- If num is any other number, return the plural form.
- Omit the third argument, plural, to use the default of the singular word + s, or supply a custom pluralized word when necessary.
- If the first argument is an object, return a function which can use the supplied dictionary to resolve the correct plural form of the word.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pluralize' to handle singular and plural forms of words
const pluralize = (val, word, plural = word + 's') => {
// Define an inner function '_pluralize' to determine the correct plural form based on the count
const _pluralize = (num, word, plural = word + 's') =>
// Check if the number is 1 or -1, return singular form, otherwise return plural form
[1, -1].includes(Number(num)) ? word : plural;
// If 'val' is an object, return a function to handle pluralization based on the key-value pairs in the object
if (typeof val === 'object') return (num, word) => _pluralize(num, word, val[word]);
// Otherwise, directly use the inner function to determine the plural form
return _pluralize(val, word, plural);
};
// Test the 'pluralize' function with different values and words
pluralize(0, 'apple'); // Output: 'apples'
pluralize(1, 'apple'); // Output: 'apple'
pluralize(2, 'apple'); // Output: 'apples'
pluralize(2, 'person', 'people'); // Output: 'people'
// Define a set of plural forms using an object
const PLURALS = {
person: 'people',
radius: 'radii'
};
// Create a specialized pluralize function based on the predefined plural forms
const autoPluralize = pluralize(PLURALS);
console.log(autoPluralize(2, 'person')); // Output: 'people'
Output:
people
Flowchart:
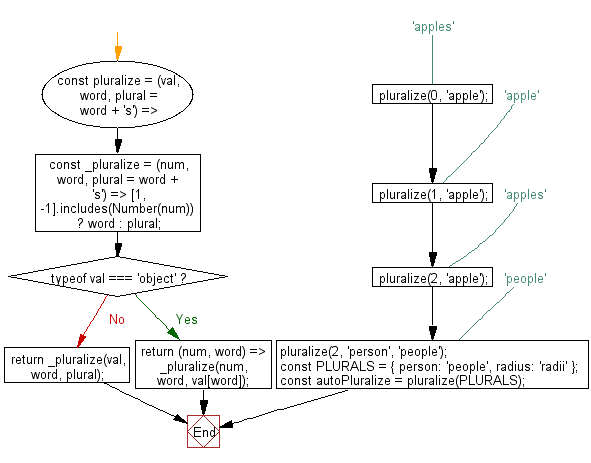
Live Demo:
See the Pen javascript-basic-exercise-163-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert a number in bytes to a human-readable string.
Next: Write a JavaScript program to perform left-to-right function composition.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-164.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics