JavaScript: Convert a number in bytes to a human-readable string
JavaScript fundamental (ES6 Syntax): Exercise-163 with Solution
Bytes to Human-Readable String
Write a JavaScript program to convert a number in bytes to a human-readable string.
Note: Use an array dictionary of units to be accessed based on the exponent.
- Use an array dictionary of units to be accessed based on the exponent.
- Use Number.prototype.toPrecision() to truncate the number to a certain number of digits.
- Return the prettified string by building it up, taking into account the supplied options and whether it is negative or not.
- Omit the second argument, precision, to use a default precision of 3 digits.
- Omit the third argument, addSpace, to add space between the number and unit by default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'prettyBytes' to convert a number of bytes into a human-readable string representation
const prettyBytes = (num, precision = 3, addSpace = true) => {
// Define an array of units in increasing order of size
const UNITS = ['B', 'KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'];
// If the absolute value of the number is less than 1, return it with the smallest unit 'B'
if (Math.abs(num) < 1) return num + (addSpace ? ' ' : '') + UNITS[0];
// Determine the appropriate unit based on the magnitude of the number
const exponent = Math.min(Math.floor(Math.log10(num < 0 ? -num : num) / 3), UNITS.length - 1);
// Calculate the value in the chosen unit with the specified precision
const n = Number(((num < 0 ? -num : num) / 1000 ** exponent).toPrecision(precision));
// Construct and return the human-readable string representation
return (num < 0 ? '-' : '') + n + (addSpace ? ' ' : '') + UNITS[exponent];
};
// Test cases
console.log(prettyBytes(1000)); // Output: 1 KB
console.log(prettyBytes(-27145424323.5821, 5)); // Output: -27.145 TB
console.log(prettyBytes(123456789, 3, false)); // Output: 123MB
Sample Output:
1 KB -27.145 GB 123MB
Pictorial Presentation:
Flowchart:
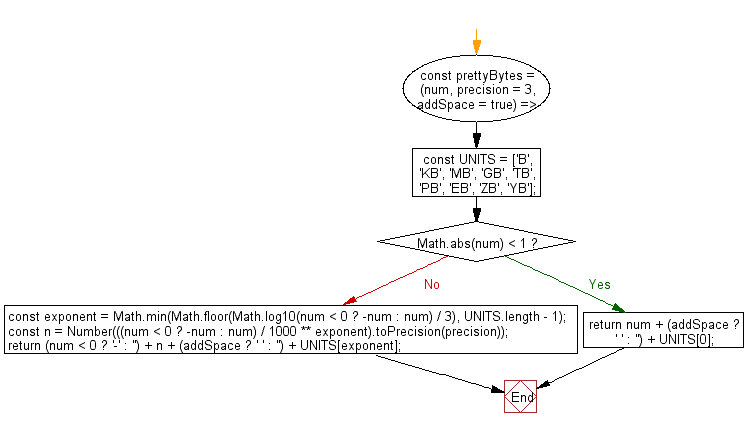
Live Demo:
See the Pen javascript-basic-exercise-163-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a byte count into a human-readable string using appropriate units (KB, MB, GB).
- Write a JavaScript function that formats a file size in bytes into a string with one decimal place and unit suffix.
- Write a JavaScript program that takes a number in bytes and outputs a localized human-readable format.
- Write a JavaScript program that converts large byte values to a readable string using logarithms to determine the unit.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert an asynchronous function to return a promise.
Next: Write a JavaScript program that will return the singular or plural form of the word based on the input number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.