JavaScript: Convert an asynchronous function to return a promise
JavaScript fundamental (ES6 Syntax): Exercise-162 with Solution
Async to Promise Conversion
Write a JavaScript program to convert an asynchronous function to return a promise.
- Use currying to return a function returning a Promise that calls the original function.
- Use the rest operator (...) to pass in all the parameters.
- Note: In Node 8+, you can use util.promisify.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'promisify' to convert a callback-based function into a promise-based function
const promisify = func => (...args) =>
// Return a new promise
new Promise((resolve, reject) =>
// Call the original function with provided arguments and a callback function
func(...args, (err, result) => (err ? reject(err) : resolve(result)))
);
// Define a callback-based function 'delay' that waits for a specified duration before invoking the callback
const delay = promisify((d, cb) => setTimeout(cb, d));
// Call 'delay' function with a delay of 2000 milliseconds and then log 'Hi!' when the promise resolves
delay(2000).then(() => console.log('Hi!')); // Promise resolves after 2s
Output:
Hi!
Flowchart:
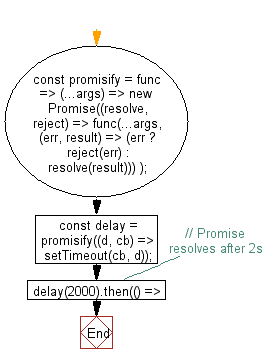
Live Demo:
See the Pen javascript-basic-exercise-162 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that wraps a callback-based asynchronous function into a promise-returning function.
- Write a JavaScript function that converts a Node.js style asynchronous function into one that returns a promise.
- Write a JavaScript program that demonstrates the conversion of setTimeout to a promise-based delay function.
- Write a JavaScript function that takes an asynchronous function with error-first callback and returns a promise for easier chaining.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to mutate the original array to filter out the values specified. Returns the removed elements.
Next: Write a JavaScript program to convert a number in bytes to a human-readable string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.