JavaScript: Mutate the original array to filter out the values specified
JavaScript fundamental (ES6 Syntax): Exercise-161 with Solution
Write a JavaScript program to mutate the original array to filter out the values specified. Returns the removed elements.
- Use Array.prototype.filter() and Array.prototype.includes() to pull out the values that are not needed.
- Set Array.prototype.length to mutate the passed in an array by resetting its length to 0.
- Use Array.prototype.push() to re-populate it with only the pulled values.
- Use Array.prototype.push() to keep track of pulled values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pullAtValue' to remove elements from an array based on specified values
const pullAtValue = (arr, pullArr) => {
// Initialize an empty array to store removed elements
let removed = [],
// Iterate over each element in the array
// If the element is included in 'pullArr', push it to the 'removed' array; otherwise, keep it
pushToRemove = arr.forEach((v, i) => (pullArr.includes(v) ? removed.push(v) : v)),
// Filter the array to remove elements that are included in 'pullArr'
mutateTo = arr.filter((v, i) => !pullArr.includes(v));
// Clear the original array
arr.length = 0;
// Push the remaining elements from the filtered array back to the original array
mutateTo.forEach(v => arr.push(v));
// Return the array of removed elements
return removed;
};
// Define an array 'myArray' containing elements
let myArray = ['a', 'b', 'c', 'd'];
// Call 'pullAtValue' function to remove elements 'b' and 'd' from 'myArray'
let pulled = pullAtValue(myArray, ['b', 'd']);
// Log the original data and pulled data
console.log('Original data');
console.log(myArray);// Output: ['a', 'c']
console.log('Pulled data');
console.log(pulled);// Output: ['b', 'd']
Output:
"Original data" ["a", "c"] "Pulled data" ["b", "d"]
Flowchart:
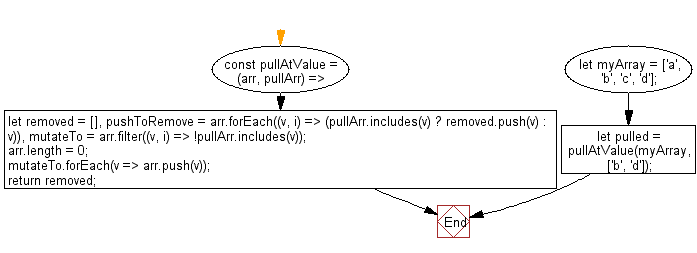
Live Demo:
See the Pen javascript-basic-exercise-161-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to mutate the original array to filter out the values specified, based on a given iterator function.
Next: Write a JavaScript program to convert an asynchronous function to return a promise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-161.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics