JavaScript: Mutate the original array to filter out the values specified, based on a given iterator function
JavaScript fundamental (ES6 Syntax): Exercise-160 with Solution
Mutate Array with Filter and Iterator
Write a JavaScript program to mutate the original array to filter out the values specified, based on a given iterator function.
- Check if the last argument provided is a function.
- Use Array.prototype.map() to apply the iterator function fn to all array elements.
- Use Array.prototype.filter() and Array.prototype.includes() to pull out the values that are not needed.
- Set Array.prototype.length to mutate the passed in an array by resetting its length to 0.
- Use Array.prototype.push() to re-populate it with only the pulled values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pullBy' that modifies the input array by removing elements based on specified criteria
// It takes a variable number of arguments:
// 1. 'arr': The array from which elements will be pulled
// 2. '...args': Additional arguments which can be either values or arrays of values to be removed
// If the last argument is a function, it will be used to extract the comparison value from elements
const pullBy = (arr, ...args) => {
// Determine the number of arguments
const length = args.length;
// Extract the last argument if it is a function, which will be used for comparison
let fn = length > 1 ? args[length - 1] : undefined;
fn = typeof fn == 'function' ? (args.pop(), fn) : undefined;
// Map the arguments to comparison values using the provided function
let argState = (Array.isArray(args[0]) ? args[0] : args).map(val => fn(val));
// Filter the array to exclude elements that match any of the comparison values
let pulled = arr.filter((v, i) => !argState.includes(fn(v)));
// Clear the original array and push the remaining elements from the filtered array
arr.length = 0;
pulled.forEach(v => arr.push(v));
};
// Define an array 'myArray' containing objects
var myArray = [{ x: 1 }, { x: 2 }, { x: 3 }, { x: 1 }];
// Call 'pullBy' function to remove elements from 'myArray' based on specified criteria
pullBy(myArray, [{ x: 1 }, { x: 3 }], o => o.x);
// Log the modified 'myArray'
console.log(myArray); // Output: [{ x: 2 }]
Output:
[{"x":2}]
Visual Presentation:
Flowchart:
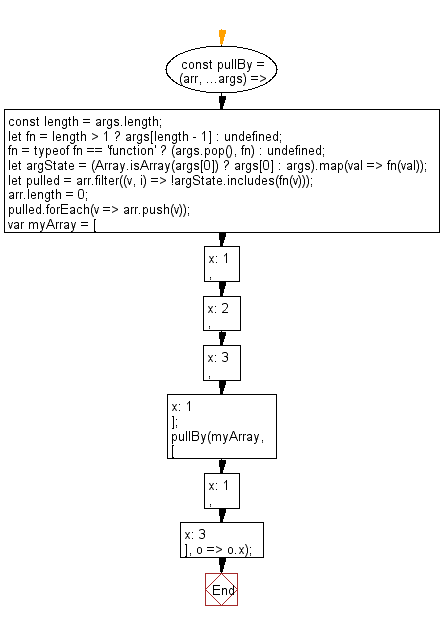
Live Demo:
See the Pen javascript-basic-exercise-160-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get an array of lines from the specified file.
Next: Write a JavaScript program to mutate the original array to filter out the values specified. Returns the removed elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics