JavaScript: Create a function that invokes the provided function with its arguments arranged according to the specified indexes
JavaScript: Exercise-158 with Solution
Write a JavaScript program to create a function that invokes the provided function with its arguments arranged according to the specified indexes.
- Use Array.prototype.map() to reorder arguments based on indexes.
- Use the spread operator (...) to pass the transformed arguments to fn.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'rearg' that rearranges the arguments passed to another function based on specified indexes
// It takes two parameters:
// 1. 'fn': The function whose arguments need to be rearranged
// 2. 'indexes': An array of indexes specifying the new order of arguments
const rearg = (fn, indexes) =>
// Return a new function that takes any number of arguments ('...args')
(...args) =>
// Call the original function 'fn' with rearranged arguments using 'indexes' array
fn(...indexes.map(i => args[i]));
// Define a function 'rearged' which is the result of rearranging the arguments of a given function
var rearged = rearg(
// Original function that takes three arguments and returns an array of those arguments
function(a, b, c) {
return [a, b, c];
},
// Array specifying the new order of arguments
[2, 0, 1]
);
// Call the 'rearged' function with three arguments 'b', 'c', and 'a'
console.log(rearged('b', 'c', 'a')); // Logs ['a', 'b', 'c'], as the arguments are rearranged
Output:
["a","b","c"]
Visual Presentation:
Flowchart:
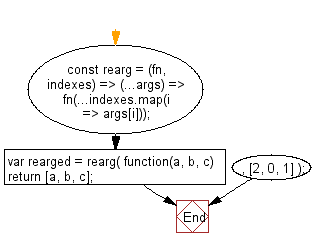
Live Demo:
See the Pen javascript-basic-exercise-158-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to redirect to a specified URL.
Next: Write a JavaScript program to get an array of lines from the specified file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-158.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics