JavaScript: Redirect to a specified URL
JavaScript: Exercise-157 with Solution
Redirect to URL
Write a JavaScript program to redirect to a specified URL.
- Use Window.location.href or Window.location.replace() to redirect to url.
- Pass a second argument to simulate a link click (true - default) or an HTTP redirect (false).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'redirect' that redirects the browser to a specified URL
// If 'asLink' is true, it uses the current window's location to navigate to the URL
// If 'asLink' is false, it replaces the current window's location with the specified URL
const redirect = (url, asLink = true) =>
// Check if 'asLink' is true
asLink
// If true, assign the specified URL to the current window's location href, causing navigation to the URL
? (window.location.href = url)
// If false, replace the current window's location with the specified URL
: window.location.replace(url);
// Call the 'redirect' function with a URL to redirect the browser to
redirect('https://google.com'); // Redirects the browser to 'https://google.com'
Flowchart:
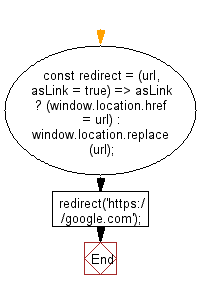
Live Demo:
See the Pen javascript-basic-exercise-156-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that performs a delayed redirect to a specified URL using setTimeout.
- Write a JavaScript function that redirects the browser to a new URL and logs the current URL before leaving.
- Write a JavaScript program that conditionally redirects to a different URL based on query parameters.
- Write a JavaScript program that checks if a user is authenticated and redirects them to a login page if not.
Go to:
PREV : Array Successive Reduce Values.
NEXT : Rearrange Function Arguments.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.