JavaScript: Round a number to a specified amount of digits
JavaScript fundamental (ES6 Syntax): Exercise-152 with Solution
Round Number to Digits
Write a JavaScript program to round a number to a specified amount of digits.
- Use Math.round() and template literals to round the number to the specified number of digits.
- Omit the second argument, decimals, to round to an integer.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'round' that rounds a number 'n' to the specified number of 'decimals'
const round = (n, decimals = 0) =>
// Convert 'n' to a string in scientific notation with the desired number of decimals
Number(`${Math.round(`${n}e${decimals}`)}e-${decimals}`);
// Test the 'round' function with different inputs
console.log(round(1.005, 2)); // Output: 1.01
console.log(round(1.05, 2)); // Output: 1.05
console.log(round(1.0005, 2)); // Output: 1
Output:
1.01 1.05 1
Visual Presentation:
Flowchart:
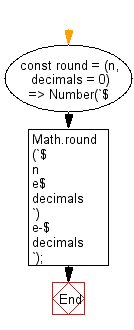
Live Demo:
See the Pen javascript-basic-exercise-152-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to run a function in a separate thread by using a Web Worker, allowing long running functions to not block the UI.
Next: Write a JavaScript program to reverse the order of the characters in the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics