JavaScript: Run a given array of promises in series
JavaScript fundamental (ES6 Syntax): Exercise-150 with Solution
Run Promises in Series
Write a JavaScript program to run a given array of promises in series.
- Use Array.prototype.reduce() to create a promise chain, where each promise returns the next promise when resolved.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the runPromisesInSeries function
const runPromisesInSeries = ps => ps.reduce((p, next) => p.then(next), Promise.resolve());
// Define a delay function that returns a promise resolving after a specified time
const delay = d => new Promise(r => setTimeout(r, d));
// Executes each promise sequentially, taking a total of 3 seconds to complete
runPromisesInSeries([() => delay(1000), () => delay(2000)]);
Flowchart:
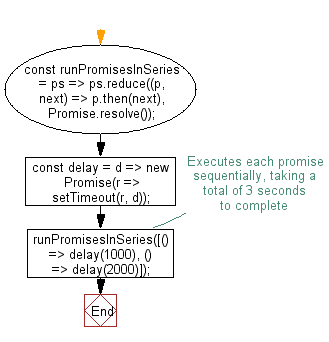
For more Practice: Solve these Related Problems:
- Write a JavaScript program that runs an array of promise-returning functions in series, ensuring each promise resolves before starting the next.
- Write a JavaScript function that chains promises sequentially using Array.reduce to process asynchronous tasks one after another.
- Write a JavaScript program that executes promises in order and collects their results into an array, handling errors gracefully.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a random element from an array.
Next: Write a JavaScript program to run a function in a separate thread by using a Web Worker, allowing long running functions to not block the UI.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics