JavaScript: Randomize the order of the values of an array, returning a new array
JavaScript fundamental (ES6 Syntax): Exercise-145 with Solution
Randomize Array Values
Write a JavaScript program to randomize the order of array values, returning an updated array.
- Use the Fisher-Yates algorithm to reorder the elements of the array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the shuffle function
const shuffle = ([...arr]) => {
let m = arr.length;
// Iterate over the array elements
while (m) {
// Pick a random index
const i = Math.floor(Math.random() * m--);
// Swap the current element with a random element
[arr[m], arr[i]] = [arr[i], arr[m]];
}
// Return the shuffled array
return arr;
};
// Test the shuffle function with an array
const foo = [1, 2, 3];
console.log(shuffle(foo)); // Output: A shuffled version of the input array
Output:
[2,1,3]
Visual Presentation:
Flowchart:
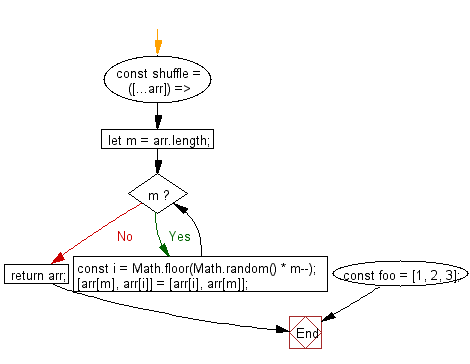
Live Demo:
See the Pen javascript-basic-exercise-145-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that randomizes the order of elements in an array using the Fisher-Yates shuffle algorithm.
- Write a JavaScript function that shuffles an array in place and returns the randomized array.
- Write a JavaScript program that creates a new array with the elements of the original array in a random order.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get an array of elements that appear in both arrays.
Next: Write a JavaScript program to create a shallow clone of an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.