JavaScript: Get the lowest index at which value should be inserted into array in order to maintain its sort order
JavaScript fundamental (ES6 Syntax): Exercise-142 with Solution
Lowest Index for Sorted Insertion
Write a JavaScript program to get the lowest index at which values should be inserted into an array in order to maintain its sorting order.
Note: Check if the array is sorted in descending order (loosely).
- Loosely check if the array is sorted in descending order.
- Use Array.prototype.findIndex() to find the appropriate index where the element should be inserted.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'sortedIndex' function
const sortedIndex = (arr, n) => {
// Determine if the array is sorted in descending order
const isDescending = arr[0] > arr[arr.length - 1];
// Find the index where 'n' should be inserted into the sorted array
const index = arr.findIndex(el => (isDescending ? n >= el : n <= el));
// Return the index or the length of the array if 'n' is greater than all elements
return index === -1 ? arr.length : index;
};
// Test the 'sortedIndex' function
console.log(sortedIndex([5, 3, 2, 1], 4)); // Output: 1
console.log(sortedIndex([30, 50], 40)); // Output: 1
Output:
1 1
Flowchart:
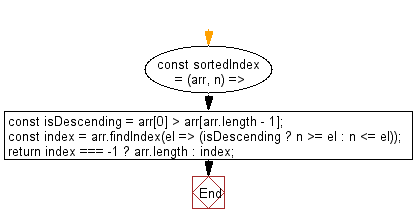
Live Demo:
See the Pen javascript-basic-exercise-142-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the lowest index at which a value can be inserted into a sorted array while preserving order.
- Write a JavaScript function that iterates over a sorted array to return the first suitable insertion index for a given number.
- Write a JavaScript program that employs a binary search to efficiently find the lowest valid insertion index in an array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the highest index at which value should be inserted into array in order to maintain its sort order.
Next: Write a JavaScript program to sort the characters of a string Alphabetically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics