JavaScript: Get the highest index at which value should be inserted into array in order to maintain its sort order
JavaScript fundamental (ES6 Syntax): Exercise-141 with Solution
Write a JavaScript program to get the highest index at which value should be inserted into an array in order to maintain its sort order.
- Loosely check if the array is sorted in descending order.
- Use Array.prototype.reverse() and Array.prototype.findIndex() to find the appropriate last index where the element should be inserted.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'sortedLastIndex' function
const sortedLastIndex = (arr, n) => {
// Determine if the array is sorted in descending order
const isDescending = arr[0] > arr[arr.length - 1];
// Find the index where 'n' should be inserted into the sorted array
const index = arr
.reverse() // Reverse the array to search from the end
.findIndex(el => (isDescending ? n <= el : n >= el)); // Find the index based on sorting order
// Return the index after adjusting for the reversed array and considering array length
return index === -1 ? 0 : arr.length - index;
};
// Test the 'sortedLastIndex' function
console.log(sortedLastIndex([10, 20, 30, 30, 40], 30)); // Output: 4
Output:
4
Flowchart:
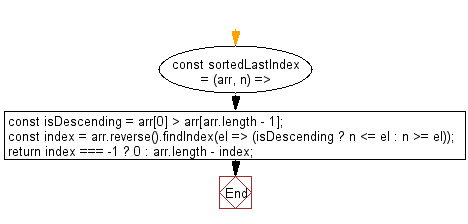
Live Demo:
See the Pen javascript-basic-exercise-141-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the highest index at which value should be inserted into array in order to maintain its sort order, based on a provided iterator function.
Next: Write a JavaScript program to get the lowest index at which value should be inserted into array in order to maintain its sort order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-141.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics