JavaScript : Get the highest index at which value should be inserted into array in order to maintain its sort order, based on a provided iterator function
JavaScript: Exercise-140 with Solution
Highest Index for Sorted Insertion (With Iterator)
Write a JavaScript program to get the highest index at which value should be inserted into an array in order to maintain its sort order. This is based on a provided iterator function.
- Loosely check if the array is sorted in descending order.
- Use Array.prototype.map() to apply the iterator function to all elements of the array.
- Use Array.prototype.reverse() and Array.prototype.findIndex() to find the appropriate last index where the element should be inserted, based on the provided iterator function.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'sortedLastIndexBy' function
const sortedLastIndexBy = (arr, n, fn) => {
// Determine if the array is sorted in descending order based on the function's result
const isDescending = fn(arr[0]) > fn(arr[arr.length - 1]);
// Get the value of 'n' after applying the function 'fn'
const val = fn(n);
// Find the index where 'val' should be inserted into the sorted array
const index = arr
.map(fn)
.reverse() // Reverse the array to search from the end
.findIndex(el => (isDescending ? val <= el : val >= el)); // Find the index based on sorting order
// Return the index after adjusting for the reversed array and considering array length
return index === -1 ? 0 : arr.length - index;
};
// Test the 'sortedLastIndexBy' function
console.log(sortedLastIndexBy([{ x: 4 }, { x: 5 }], { x: 4 }, o => o.x)); // Output: 1
Output:
1
Flowchart:
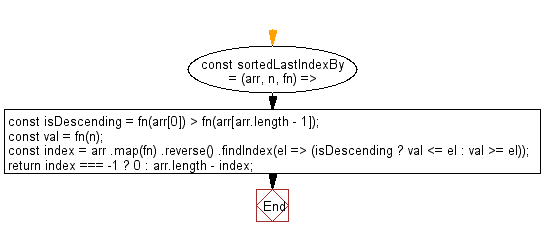
Live Demo:
See the Pen javascript-basic-exercise-140-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines the highest index at which a value should be inserted into a sorted array using an iterator function.
- Write a JavaScript function that uses a custom comparator to find the correct insertion point in a sorted array, favoring the highest index.
- Write a JavaScript program that iterates over an array with a provided function to calculate the ideal insertion index for a new value.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to split a multiline string into an array of lines.
Next: Write a JavaScript program to get the highest index at which value should be inserted into array in order to maintain its sort order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.