JavaScript: Replace the names of multiple object keys with the values provided
JavaScript fundamental (ES6 Syntax): Exercise-14 with Solution
Write a JavaScript program to replace multiple object keys' names with the values provided.
- Use Object.keys() in combination with Array.prototype.reduce() and the spread operator (...) to get the object's keys and rename them according to keysMap.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `rename_keys` that renames keys of an object based on a provided mapping.
const rename_keys = (keysMap, obj) =>
Object.keys(obj).reduce(
(acc, key) => ({
...acc,
...{ [keysMap[key] || key]: obj[key] } // Rename keys based on the keysMap, otherwise keep the original key
}),
{}
);
// Original object
const obj = { name: 'Bobo', job: 'Programmer', shoeSize: 100 };
console.log("Original Object");
console.log(obj);
console.log("-------------------------------------");
// Renaming keys according to the provided mapping
result = rename_keys({ name: 'firstName', job: 'Actor' }, obj);
// Displaying the modified object
console.log("New Object");
console.log(result);
Output:
"Original Object" [object Object] { job: "Programmer", name: "Bobo", shoeSize: 100 } "-------------------------------------" "New Object" [object Object] { Actor: "Programmer", firstName: "Bobo", shoeSize: 100 }
Flowchart:
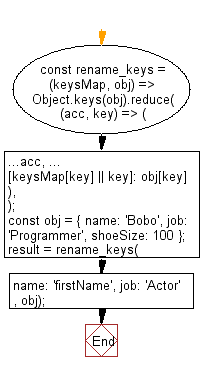
Live Demo:
See the Pen javascript-fundamental-exercise-14 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert the length of a given string in bytes.
Next: Write a JavaScript program to return the minimum-maximum value of an array, after applying the provided function to set comparing rule.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics