JavaScript: Takes a variadic function and returns a closure that accepts an array of arguments to map to the inputs of the function
JavaScript fundamental (ES6 Syntax) exercises, practice and solution: Exercise-138 with Solution
Variadic Function with Array Inputs
Write a JavaScript program that takes a variadic function and returns a closure that accepts an array of arguments to map to the inputs of the function.
Note: Use closures and the spread operator (...) to map the array of arguments to the inputs of the function.
- Use a closure and the spread operator (...) to map the array of arguments to the inputs of the function.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'spreadOver' function.
const spreadOver = fn => argsArr => fn(...argsArr);
// Create a new function 'arrayMax' that spreads its arguments and passes them to 'Math.max'.
const arrayMax = spreadOver(Math.max);
// Test the 'arrayMax' function with an array of numbers.
console.log(arrayMax([1, 2, 3])); // Output: 3
Output:
3
Visual Presentation:
Flowchart:
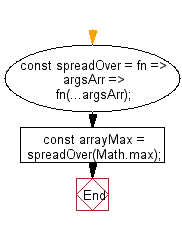
Live Demo:
See the Pen javascript-basic-exercise-138-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform stable sorting of an array, preserving the initial indexes of items when their values are the same. Do not mutate the original array, but returns a new array instead.
Next: Write a JavaScript program to split a multiline string into an array of lines.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics