JavaScript: Perform stable sorting of an array, preserving the initial indexes of items when their values are the same
JavaScript fundamental (ES6 Syntax): Exercise-137 with Solution
Stable Sort Array
Write a JavaScript program to perform stable sorting of an array, preserving the initial indexes of items when their values are the same. Returns a new array instead of mutating the original array.
- Use Array.prototype.map() to pair each element of the input array with its corresponding index.
- Use Array.prototype.sort() and a compare function to sort the list, preserving their initial order if the items compared are equal.
- Use Array.prototype.map() to convert back to the initial array items.
- Does not mutate the original array, but returns a new array instead.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'stableSort' function.
const stableSort = (arr, compare) =>
arr
.map((item, index) => ({ item, index })) // Map each item to an object containing the item and its index.
.sort((a, b) => compare(a.item, b.item) || a.index - b.index) // Sort based on the comparison function and the original indices.
.map(({ item }) => item); // Extract the sorted items from the objects and return them as an array.
// Test the 'stableSort' function with an array and a comparison function.
const arr = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
console.log(stableSort(arr, () => 0)); // Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Output:
[0,1,2,3,4,5,6,7,8,9,10]
Flowchart:
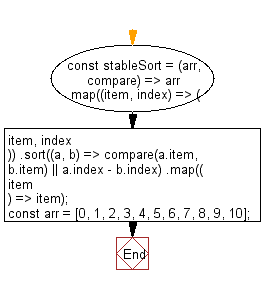
Live Demo:
See the Pen javascript-basic-exercise-137-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that performs a stable sort on an array of objects, preserving the original order of equal elements.
- Write a JavaScript function that implements a stable sorting algorithm and returns a new sorted array.
- Write a JavaScript program that sorts an array by a key and ensures that items with equal keys retain their initial positions.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to generate all permutations of a string (contains duplicates).
Next: Write a JavaScript program that takes a variadic function and returns a closure that accepts an array of arguments to map to the inputs of the function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.