JavaScript: Get the sum of the powers of all the numbers from start to end
JavaScript fundamental (ES6 Syntax): Exercise-135 with Solution
Sum of Powers in Range
Write a JavaScript program to get the sum of the powers of all the numbers from start to end (both inclusive).
- Use Array.prototype.fill() to create an array of all the numbers in the target range.
- Use Array.prototype.map() and the exponent operator (**) to raise them to power and Array.prototype.reduce() to add them together.
- Omit the second argument, power, to use a default power of 2.
- Omit the third argument, start, to use a default starting value of 1.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'sumPower' function.
const sumPower = (end, power = 2, start = 1) =>
// Create an array from 'start' to 'end', apply the power operation, and then sum the elements.
Array(end + 1 - start)
.fill(0) // Fill the array with zeros.
.map((x, i) => (i + start) ** power) // Apply the power operation to each element.
.reduce((a, b) => a + b, 0); // Sum all the elements.
// Test the 'sumPower' function with various arguments.
console.log(sumPower(10)); // Output: 385 (1^2 + 2^2 + ... + 10^2)
console.log(sumPower(10, 3)); // Output: 3025 (1^3 + 2^3 + ... + 10^3)
console.log(sumPower(10, 3, 5)); // Output: 2925 (5^3 + 6^3 + ... + 10^3)
Output:
385 3025 2925
Visual Presentation:
Flowchart:
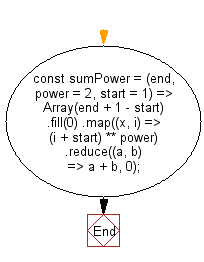
Live Demo:
See the Pen javascript-basic-exercise-135-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates the sum of the k-th powers of all integers between two given numbers.
- Write a JavaScript function that iterates from start to end and sums up each number raised to a specified power.
- Write a JavaScript program that uses a loop or reduce() to compute the sum of powers for a range of integers.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the symmetric difference between two given arrays.
Next: Write a JavaScript program to generate all permutations of a string (contains duplicates).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.