JavaScript: Get the symmetric difference between two given arrays, using a provided function as a comparator
JavaScript fundamental (ES6 Syntax): Exercise-132 with Solution
Symmetric Difference with Comparator
Write a JavaScript program to get the symmetric difference between two given arrays, using a provided function as a comparator.
- Use Array.prototype.filter() and Array.prototype.findIndex() to find the appropriate values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'symmetricDifferenceWith' function.
const symmetricDifferenceWith = (arr, val, comp) => [
...arr.filter(a => val.findIndex(b => comp(a, b)) === -1),
...val.filter(a => arr.findIndex(b => comp(a, b)) === -1)
];
// Test the 'symmetricDifferenceWith' function.
console.log(symmetricDifferenceWith(
[1, 1.2, 1.5, 3, 0],
[1.9, 3, 0, 3.9],
(a, b) => Math.round(a) === Math.round(b)
));
Output:
[1,1.2,3.9]
Flowchart:
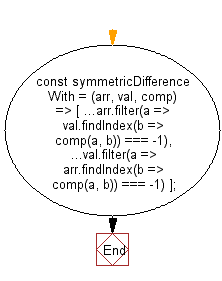
Live Demo:
See the Pen javascript-basic-exercise-132-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the symmetric difference between two arrays using a custom comparator function.
- Write a JavaScript function that returns elements that are unique to each array based on a provided equality test.
- Write a JavaScript program that iterates over two arrays and collects items that do not match between them, using a comparator.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get an array with n elements removed from the beginning from a given array.
Next: Write a JavaScript program to get the symmetric difference between two given arrays, after applying the provided function to each array element of both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.