JavaScript: Remove n elements from the end of a given array
JavaScript fundamental (ES6 Syntax) exercises, practice and solution: Exercise-130 with Solution
Remove n Elements from End
Write a JavaScript program to remove n elements from the end of a given array.
- Use Array.prototype.slice() to create a slice of the array with n elements taken from the end.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'takeRight' function.
const takeRight = (arr, n = 1) => arr.slice(arr.length - n, arr.length);
// Test the 'takeRight' function.
console.log(takeRight([1, 2, 3], 2)); // Output: [2, 3]
console.log(takeRight([1, 2, 3])); // Output: [3]
Output:
[2,3] [3]
Visual Presentation:
Flowchart:
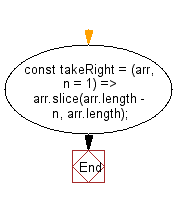
Live Demo:
See the Pen javascript-basic-exercise-130-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get removed elements from the end of a given array until the passed function returns true.
Next: Write a JavaScript program to get an array with n elements removed from the beginning from a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics