JavaScript: Get removed elements from the end of a given array until the passed function returns true
JavaScript fundamental (ES6 Syntax): Exercise-129 with Solution
Remove Elements from End Until True
Write a JavaScript program to get removed elements from the end of a given array until the passed function returns true.
- Create a reversed copy of the array, using the spread operator (...) and Array.prototype.reverse().
- Loop through the reversed copy, using a for...of loop over Array.prototype.entries() until the returned value from the function is falsy.
- Return the removed elements, using Array.prototype.slice().
- The callback function, fn, accepts a single argument which is the value of the element.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'takeRightWhile' function.
const takeRightWhile = (arr, func) => {
// Reverse the array and iterate through its keys.
for (let i of arr.reverse().keys()) {
// If the function returns false for the current value, return the slice of the original array from the end up to that index.
if (!func(arr[i])) return arr.reverse().slice(arr.length - i, arr.length);
}
// If the function returns true for all elements, return the original array.
return arr;
};
// Test the 'takeRightWhile' function.
console.log(takeRightWhile([1, 2, 3, 4], n => n < 3)); // Output: [3, 4]
Output:
[3,4]
Flowchart:
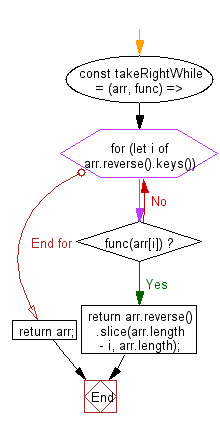
Live Demo:
See the Pen javascript-basic-exercise-129-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes elements from the end of an array until a specified function returns true.
- Write a JavaScript function that pops elements off an array until a condition is met, returning the removed elements.
- Write a JavaScript program that iterates backward over an array and removes trailing elements until a predicate is satisfied.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get removed elements of a given array until the passed function returns true.
Next: Write a JavaScript program to remove n elements from the end of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.