JavaScript: Iterate over a callback n times
JavaScript fundamental (ES6 Syntax): Exercise-127 with Solution
Iterate Callback n Times
Write a JavaScript program to iterate over a callback n times.
- Use Function.prototype.call() to call fn n times or until it returns false.
- Omit the last argument, context, to use an undefined object (or the global object in non-strict mode).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'times' function.
const times = (n, fn, context = undefined) => {
let i = 0;
while (fn.call(context, i) !== false && ++i < n) {}
};
// Define a variable to store the output.
var output = '';
// Use the 'times' function to execute a callback function for each iteration.
times(5, i => (output += i));
// Output the result.
console.log(output); // Output: "01234"
Output:
01234
Flowchart:
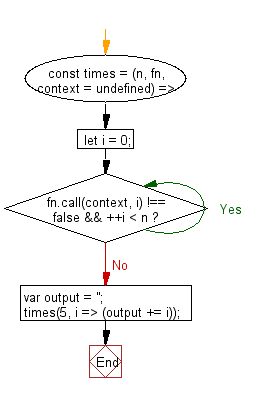
Live Demo:
See the Pen javascript-basic-exercise-127-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that executes a callback function n times and returns an array of the results.
- Write a JavaScript function that uses a loop to iterate a callback a specified number of times, collecting outputs.
- Write a JavaScript program that simulates a for loop by invoking a function repeatedly for a given count.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a specified currency formatting from a given number.
Next: Write a JavaScript program to get removed elements of an given array until the passed function returns true.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.