JavaScript: Reduce a given Array-like into a value hash
JavaScript fundamental (ES6 Syntax): Exercise-124 with Solution
Reduce Array-Like to Hash Map
Write a JavaScript program to reduce a given Array-like into a value hash (keyed data store).
Note: Given an Iterable or Array-like structure, call Array.prototype.reduce.call() on the provided object to step over it and return an Object, keyed by the reference value.
- Given an iterable object or array-like structure, call Array.prototype.reduce.call() on the provided object to step over it and return an Object, keyed by the reference value.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'toHash' function.
const toHash = (object, key) =>
Array.prototype.reduce.call(
object,
(acc, data, index) => ((acc[!key ? index : data[key]] = data), acc),
{}
);
// Test the 'toHash' function with sample inputs.
toHash([4, 3, 2, 1]);
toHash([{ a: 'label' }], 'a');
// A more in-depth example
let users = [{ id: 1, first: 'Jon' }, { id: 2, first: 'Joe' }, { id: 3, first: 'Moe' }];
let managers = [{ manager: 1, employees: [2, 3] }];
managers.forEach(
manager =>
(manager.employees = manager.employees.map(function(id) {
return this[id];
}, toHash(users, 'id')))
);
// Output the result.
console.log(managers); // Output: [ { manager: 1, employees: [ { id: 2, first: 'Joe' }, { id: 3, first: 'Moe' } ] } ]
Output:
[{"manager":1,"employees":[{"id":2,"first":"Joe"},{"id":3,"first":"Moe"}]}]
Flowchart:
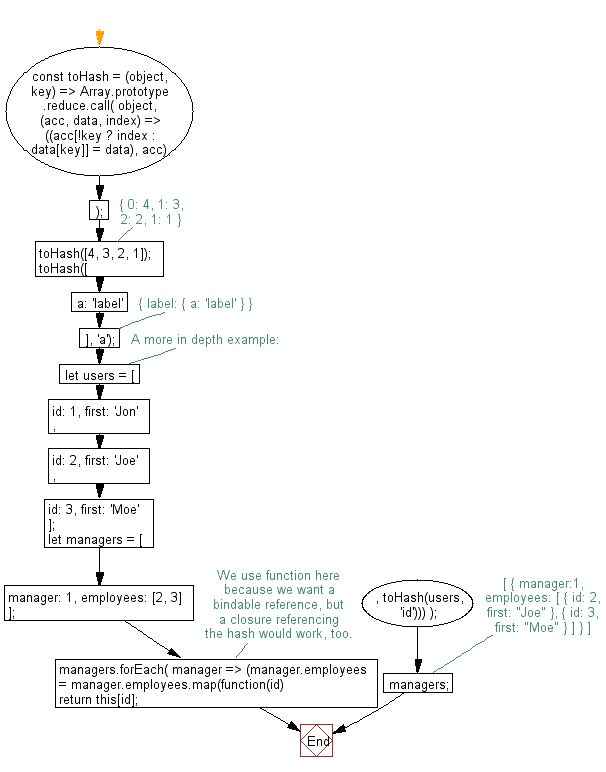
Live Demo:
See the Pen javascript-basic-exercise-124-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts an array-like object into a hash map using a reducer function.
- Write a JavaScript function that iterates over an array-like structure and builds an object keyed by each element’s index.
- Write a JavaScript program that transforms an array into an object by mapping each element to a key-value pair via a callback.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert a string to kebab case.
Next: Write a JavaScript program to convert a float-point arithmetic to the Decimal mark form and It will make a comma separated string from a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics