JavaScript: Add an ordinal suffix to a number
JavaScript fundamental (ES6 Syntax): Exercise-122 with Solution
Add Ordinal Suffix to Number
Write a JavaScript program to add an ordinal suffix to a number.
- Use the modulo operator (%) to find values of single and tens digits.
- Find which ordinal pattern digits match.
- If digit is found in teens pattern, use teens ordinal.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'toOrdinalSuffix' function.
const toOrdinalSuffix = num => {
const int = parseInt(num),
digits = [int % 10, int % 100],
ordinals = ['st', 'nd', 'rd', 'th'],
oPattern = [1, 2, 3, 4],
tPattern = [11, 12, 13, 14, 15, 16, 17, 18, 19];
return oPattern.includes(digits[0]) && !tPattern.includes(digits[1])
? int + ordinals[digits[0] - 1]
: int + ordinals[3];
};
// Test the 'toOrdinalSuffix' function with sample inputs.
console.log(toOrdinalSuffix('1')); // Output: 1st
console.log(toOrdinalSuffix('4')); // Output: 4th
console.log(toOrdinalSuffix('50')); // Output: 50th
console.log(toOrdinalSuffix('123')); // Output: 123rd
Output:
1st 4th 50th 123rd
Flowchart:
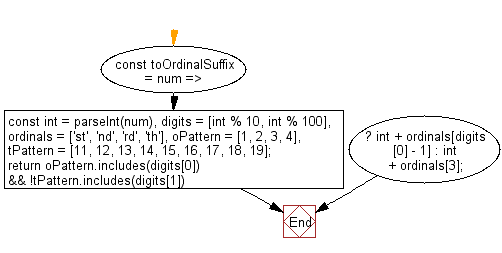
Live Demo:
See the Pen javascript-basic-exercise-122-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that appends the appropriate ordinal suffix (st, nd, rd, th) to a given number.
- Write a JavaScript function that handles edge cases for ordinal suffixes, such as 11th, 12th, and 13th.
- Write a JavaScript program that converts a number to a string with its ordinal indicator based on its last digit.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert a value to a safe integer.
Next: Write a JavaScript program to convert a string to kebab case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics