JavaScript: Convert a string to snake case
JavaScript fundamental (ES6 Syntax): Exercise-120 with Solution
String to Snake Case
Write a JavaScript program to convert a string to snake case.
Note: Break the string into words and combine them adding _ as a separator, using a regexp.
- Use String.prototype.match() to break the string into words using an appropriate regexp.
- Use Array.prototype.map(), Array.prototype.slice(), Array.prototype.join() and String.prototype.toLowerCase() to combine them, adding _ as a separator.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'toSnakeCase' function.
const toSnakeCase = str =>
str &&
str
.match(/[A-Z]{2,}(?=[A-Z][a-z]+[0-9]*|\b)|[A-Z]?[a-z]+[0-9]*|[A-Z]|[0-9]+/g) // Match words
.map(x => x.toLowerCase()) // Convert to lowercase
.join('_'); // Join words with underscores
// Test the 'toSnakeCase' function with sample inputs.
console.log(toSnakeCase('camelCase')); // Output: camel_case
console.log(toSnakeCase('some text')); // Output: some_text
console.log(toSnakeCase('some-mixed_string With spaces_underscores-and-hyphens')); // Output: some_mixed_string_with_spaces_underscores_and_hyphens
console.log(toSnakeCase('AllThe-small Things')); // Output: all_the_small_things
console.log(toSnakeCase('IAmListeningToFMWhileLoadingDifferentURLOnMyBrowserAndAlsoEditingSomeXMLAndHTML')); // Output: i_am_listening_to_fm_while_loading_different_url_on_my_browser_and_also_editing_some_xml_and_html
Output:
camel_case some_text some_mixed_string_with_spaces_underscores_and_hyphens all_the_small_things i_am_listening_to_fm_while_loading_different_url_on_my_browser_and_also_editing_some_xml_and_html
Visual Presentation:
Flowchart:
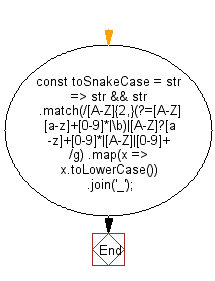
Live Demo:
See the Pen javascript-basic-exercise-120-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a given string to snake_case by replacing spaces and capital letters appropriately.
- Write a JavaScript function that transforms a camelCase or PascalCase string into snake_case.
- Write a JavaScript program that normalizes a string to lowercase and replaces all non-alphanumeric characters with underscores.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create tomorrow's date in a string representation.
Next: Write a JavaScript program to convert a value to a safe integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.