JavaScript: Create tomorrow's date in a string representation
JavaScript fundamental (ES6 Syntax): Exercise-119 with Solution
String for Tomorrow's Date
Write a JavaScript program to create tomorrow's date in a string representation.
- Use new Date() to get the current date.
- Increment it by one using Date.prototype.getDate() and set the value to the result using Date.prototype.setDate().
- Use Date.prototype.toISOString() to return a string in yyyy-mm-dd format.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'tomorrow' function.
const tomorrow = (long = false) => {
// Create a new Date object representing tomorrow's date.
let t = new Date();
t.setDate(t.getDate() + 1);
// Format the date string in YYYY-MM-DD format.
const ret = `${t.getFullYear()}-${String(t.getMonth() + 1).padStart(2, '0')}-${String(t.getDate()).padStart(2, '0')}`;
// Return the date string with or without the time component.
return !long ? ret : `${ret}T00:00:00`;
};
// Example usage: Get tomorrow's date.
console.log(tomorrow());
console.log(tomorrow(true));
Output:
2018-08-17 2018-08-17T00:00:00
Visual Presentation:
Flowchart:
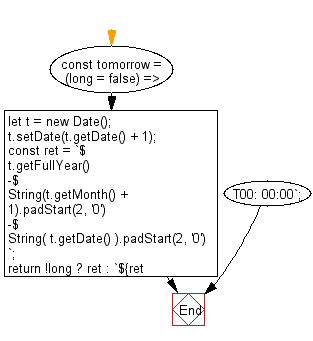
Live Demo:
See the Pen javascript-basic-exercise-119-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes tomorrow’s date and returns it as a formatted string.
- Write a JavaScript function that calculates the next day and converts it to a human-readable date string.
- Write a JavaScript program that adds one day to the current date and formats the result in "YYYY-MM-DD" format.
Go to:
PREV : Reduce Object with Accumulator Function.
NEXT : String to Snake Case.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.