JavaScript: Apply a function against an accumulator and each key in the object
JavaScript fundamental (ES6 Syntax): Exercise-118 with Solution
Reduce Object with Accumulator Function
Write a JavaScript program to apply a function against an accumulator and each key in the object (from left to right).
- Use Object.keys() to iterate over each key in the object.
- Use Array.prototype.reduce() to apply the specified function against the given accumulator.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'transform' function.
const transform = (obj, fn, acc) =>
// Use Object.keys to iterate over the keys of the object.
Object.keys(obj).reduce(
// Use reduce to apply the transformation function to each key-value pair.
(a, k) => fn(a, obj[k], k, obj), // Call the transformation function with accumulator, value, key, and object.
acc // Set the initial value of the accumulator.
);
// Example usage: Transform an object using a custom function.
console.log(transform(
{ a: 1, b: 2, c: 1 }, // Input object
// Transformation function: Group keys by their corresponding values.
(r, v, k) => {
// Initialize the array for the current value if it doesn't exist in the accumulator.
(r[v] || (r[v] = [])).push(k);
return r; // Return the updated accumulator.
},
{} // Initial value of the accumulator.
));
// Output: { '1': ['a', 'c'], '2': ['b'] }
Output:
{"1":["a","c"],"2":["b"]}
Flowchart:
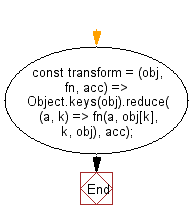
Live Demo:
See the Pen javascript-basic-exercise-118-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that iterates over an object’s keys and reduces it to a single value using an accumulator function.
- Write a JavaScript function that applies a reducer to an object, combining its values into a summary value.
- Write a JavaScript program that mimics Array.reduce() for objects by processing each key-value pair sequentially.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to truncate a string up to a specified length.
Next: Write a JavaScript program to create tomorrow's date in a string representation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.