JavaScript: Truncate a string up to a specified length
JavaScript fundamental (ES6 Syntax): Exercise-117 with Solution
Write a JavaScript program to truncate a string up to a specified length.
Note: Determine if the string's length is greater than num. Return the string truncated to the desired length, with '...' appended to the end or the original string.
- Determine if String.prototype.length is greater than num.
- Return the string truncated to the desired length, with '...' appended to the end or the original string.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'truncateString' function.
const truncateString = (str, num) =>
// Check if the length of the string is greater than the specified number.
str.length > num ?
// If so, truncate the string to the specified length and add ellipsis if necessary.
str.slice(0, num > 3 ? num - 3 : num) + '...'
// If not, return the original string.
: str;
// Example usage: Truncate a string if it exceeds a certain length.
console.log(truncateString('boomerang', 7));
// Output: "boom..."
Output:
boom...
Visual Presentation:
Flowchart:
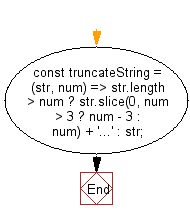
Live Demo:
See the Pen javascript-basic-exercise-117-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if the predicate (second argument) is truthy on all elements of a collection (first argument).
Next: Write a JavaScript program to apply a function against an accumulator and each key in the object (from left to right).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-117.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics