JavaScript: Uncurry a function up to depth n
JavaScript fundamental (ES6 Syntax): Exercise-114 with Solution
Uncurry Function to Depth n
Write a JavaScript program to uncurry a function up to depth n.
- Return a variadic function.
- Use Array.prototype.reduce() on the provided arguments to call each subsequent curry level of the function.
- If the length of the provided arguments is less than n throw an error.
- Otherwise, call fn with the proper amount of arguments, using Array.prototype.slice(0, n).
- Omit the second argument, n, to uncurry up to depth 1.
Sample Solution:
JavaScript Code:
Output:
6
Visual Presentation:
Flowchart:
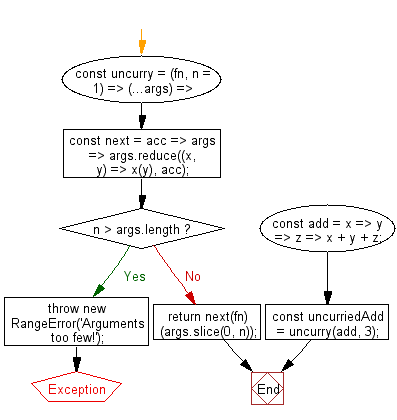
Live Demo:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that uncurry a nested function structure up to a specified depth n.
- Write a JavaScript function that transforms a curried function into a function accepting multiple arguments at once.
- Write a JavaScript program that flattens a curried function's parameters by recursively combining its nested functions.
Go to:
PREV : Unescape HTML Characters.
NEXT : Single-Argument Function.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.