JavaScript: Unescape escaped HTML characters
JavaScript fundamental (ES6 Syntax): Exercise-113 with Solution
Unescape HTML Characters
Write a JavaScript program to unescape escaped HTML characters.
- Use String.prototype.replace() with a regexp that matches the characters that need to be unescaped.
- Use the function's callback to replace each escaped character instance with its associated unescaped character using a dictionary (object).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'unescapeHTML' function.
const unescapeHTML = str =>
// Use 'String.replace' with a regular expression to replace HTML escape characters.
str.replace(
/&|<|>|'|"/g,
// Use an object to map escape characters to their corresponding symbols.
tag =>
({
'&': '&',
'<': '<',
'>': '>',
'\'': '\'',
'"': '"'
}[tag] || tag)
);
// Test the 'unescapeHTML' function with a sample HTML string.
console.log(unescapeHTML('<a href="#">Me & you</a>'));
// Output: '<a href="#">Me & you</a>'
Output:
"<a href=\"#\">Me & you</a>"
Flowchart:
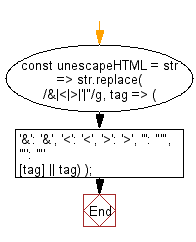
Live Demo:
See the Pen javascript-basic-exercise-113-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts escaped HTML entities in a string back to their original characters.
- Write a JavaScript function that replaces common HTML escape sequences (e.g., <, >) with their literal counterparts.
- Write a JavaScript program that processes a string containing HTML entities and returns the unescaped text.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to unflatten an object with the paths for keys.
Next: Write a JavaScript program to uncurry a function up to depth n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.