JavaScript: Build an array, using an iterator function and an initial seed value
JavaScript fundamental (ES6 Syntax): Exercise-111 with Solution
Build Array with Iterator and Seed
Write a JavaScript program to build an array, using an iterator function and an initial seed value.
- Use a while loop and Array.prototype.push() to call the function repeatedly until it returns false.
- The iterator function accepts one argument (seed) and must always return an array with two elements ([value, nextSeed]) or false to terminate.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'unfold' function.
const unfold = (fn, seed) => {
// Initialize an empty array to store the result.
let result = [];
// Initialize the value 'val' with an array containing 'null' and the 'seed'.
let val = [null, seed];
// Use a 'while' loop to iterate until the condition provided by the 'fn' function is false.
while ((val = fn(val[1]))) {
// Push the first element of the 'val' array (generated by the 'fn' function) to the 'result' array.
result.push(val[0]);
}
// Return the 'result' array.
return result;
};
// Define the function 'f' which takes a number 'n' and returns an array containing two elements:
// The negative of 'n' and 'n + 10'.
const f = n => (n > 50 ? false : [-n, n + 10]);
// Test the 'unfold' function with the 'f' function and an initial seed of 10.
console.log(unfold(f, 10)); // Output: [-10, -20, -30, -40, -50]
Output:
[-10,-20,-30,-40,-50]
Flowchart:
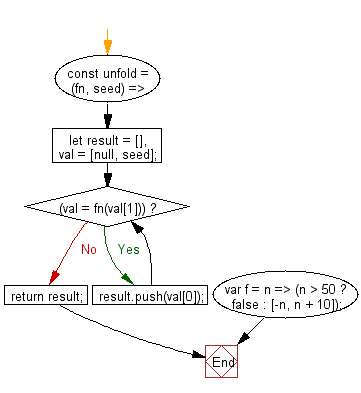
Live Demo:
See the Pen javascript-basic-exercise-111-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that builds an array by repeatedly applying an iterator function starting from a seed value.
- Write a JavaScript function that uses a for loop to generate an array of values based on an initial seed and an updating function.
- Write a JavaScript program that constructs an array of a specified length by iteratively computing each element from the previous one.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get every element that exists in any of the two arrays once.
Next: Write a JavaScript program to unflatten an object with the paths for keys.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.