JavaScript: Get every element that exists in any of the two arrays once
JavaScript fundamental (ES6 Syntax): Exercise-110 with Solution
Write a JavaScript program to get every element in any of the two arrays at once.
Note: Create a Set with all values of a and b and convert to an array.
- Create a new Set() with all values of a and b and convert it to an array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'union' to return the union of two arrays.
const union = (a, b) =>
// Use the spread operator (...) to concatenate the arrays 'a' and 'b'.
// Use the 'Set' object to create a new set from the concatenated array, removing duplicates.
// Convert the set back to an array using 'Array.from' to get the union of the two arrays.
Array.from(new Set([...a, ...b]));
// Test the 'union' function with examples.
console.log(union([1, 2, 3], [4, 3, 2])); // Output: [1, 2, 3, 4]
console.log(union([1, 2, 3], [1, 2, 3])); // Output: [1, 2, 3]
Output:
[1,2,3,4] [1,2,3]
Visual Presentation:
Flowchart:
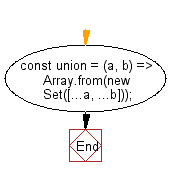
Live Demo:
See the Pen javascript-basic-exercise-110-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the nth element of an given array.
Next: Write a JavaScript program to build an array, using an iterator function and an initial seed value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-110.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics