JavaScript : Get the nth element of a given array
JavaScript fundamental (ES6 Syntax): Exercise-109 with Solution
Get nth Element from Array
Write a JavaScript program to get the nth element of a given array.
- Use Array.prototype.slice() to get an array containing the nth element at the first place.
- If the index is out of bounds, return undefined.
- Omit the second argument, n, to get the first element of the array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'nthElement' to return the nth element of an array.
const nthElement = (arr, n = 0) =>
// Use ternary operator to check if 'n' is greater than 0.
// If true, use 'slice' to extract the nth element from 'arr' and return it.
// If false, use 'slice' to extract the nth element from the end of 'arr' and return it.
(n > 0 ? arr.slice(n, n + 1) : arr.slice(n))[0];
// Test the 'nthElement' function with examples.
console.log(nthElement(['a', 'b', 'c'], 1)); // Output: 'b'
console.log(nthElement(['a', 'b', 'b'], -3)); // Output: 'a'
Output:
b a
Visual Presentation:
Flowchart:
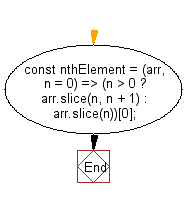
Live Demo:
See the Pen javascript-basic-exercise-109-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the element at the nth index of an array, handling out-of-bound indexes gracefully.
- Write a JavaScript function that retrieves the nth element of an array using array destructuring or slicing.
- Write a JavaScript program that accepts a number n and an array, then returns the nth element, defaulting to the last element if n is negative.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get all unique values of a array, based on a provided comparator function.
Next: Write a JavaScript program to get every element that exists in any of the two arrays once.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.