JavaScript: Create an array of elements, ungrouping the elements in an array produced by zip and applying the provided function
JavaScript fundamental (ES6 Syntax): Exercise-106 with Solution
Ungroup Array with Function
Write a JavaScript program to create an array of elements, ungrouping the elements in an array produced by zip and applying the provided function.
- Use Math.max(), Function.prototype.apply() to get the longest subarray in the array, Array.prototype.map() to make each element an array.
- Use Array.prototype.reduce() and Array.prototype.forEach() to map grouped values to individual arrays.
- Use Array.prototype.map() and the spread operator (...) to apply fn to each individual group of elements.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'unzipWith' to unzip an array of arrays and apply a function to each corresponding element.
const unzipWith = (arr, fn) =>
// Reduce the input array 'arr' to unzip the arrays and apply the function 'fn'.
arr.reduce(
// For each value in the array 'arr', update the accumulator 'acc'.
(acc, val) => (
// For each value 'v' in 'val', push it to the corresponding array in 'acc'.
val.forEach((v, i) => acc[i].push(v)),
// Return the updated accumulator.
acc
),
// Initialize the accumulator as an array of arrays with lengths equal to the maximum length of the input arrays.
Array.from({
length: Math.max(...arr.map(x => x.length))
}).map(x => [])
)
// Map over each array in the unzipped arrays and apply the function 'fn' to their elements.
.map(val => fn(...val));
// Test the 'unzipWith' function with an example.
console.log(unzipWith([[1, 10, 100], [2, 20, 200]], (...args) => args.reduce((acc, v) => acc + v, 0))); // Output: [3, 30, 300]
Output:
[3,30,300]
Visual Presentation:
Flowchart:
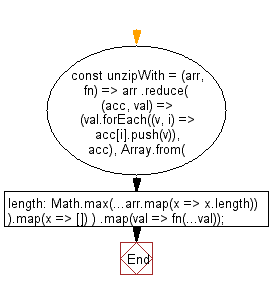
Live Demo:
See the Pen javascript-basic-exercise-106-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes a nested array produced by a zip function and flattens it using a provided ungrouping function.
- Write a JavaScript function that reverses the grouping of an array of arrays, returning a single flattened array.
- Write a JavaScript program that applies a mapping function to ungroup elements from a nested array into a single-level array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that return true if the given value is a number, false otherwise.
Next: Write a JavaScript program to get all unique values (form the right side of the array) of an array, based on a provided comparator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.