JavaScript: Return true if the given value is a number, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-105 with Solution
Check if Value is a Number
Write a JavaScript program that returns true if the given value is a number, false otherwise.
- Use parseFloat() to try to convert n to a number.
- Use !Number.isNaN() to check if num is a number.
- Use Number.isFinite() to check if num is finite.
- Use Number() and the loose equality operator (==) to check if the coercion holds.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'validateNumber' to check if a value is a valid number.
const validateNumber = n =>
!isNaN(parseFloat(n)) && // Check if it's a valid number when parsed as a float.
isFinite(n) && // Check if it's a finite number.
Number(n) == n; // Check if converting back to a number gives the same value.
// Test the 'validateNumber' function with different inputs.
console.log(validateNumber('200')); // Output: true (valid number)
console.log(validateNumber('10')); // Output: true (valid number)
console.log(validateNumber('abc')); // Output: false (not a valid number)
Output:
true true false
Visual Presentation:
Flowchart:
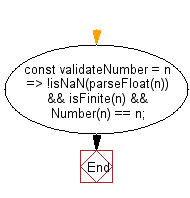
Live Demo:
See the Pen javascript-basic-exercise-105-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a given value is a number and false otherwise, using typeof and isNaN checks.
- Write a JavaScript function that validates whether an input is a finite number, excluding NaN and Infinity.
- Write a JavaScript program that determines if a value is numeric by testing its conversion to a number and verifying its type.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to test a value, x, against a predicate function. If true, return fn(x). Else, return x.
Next: Write a JavaScript program to create an array of elements, ungrouping the elements in an array produced by zip and applying the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.