JavaScript: Convert a given string into an array of words
JavaScript fundamental (ES6 Syntax): Exercise-103 with Solution
String to Array of Words
Write a JavaScript program to convert a given string into an array of words.
- Use String.prototype.split() with a supplied pattern (defaults to non-alpha as a regexp) to convert to an array of strings.
- Use Array.prototype.filter() to remove any empty strings.
- Omit the second argument, pattern, to use the default regexp.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'words' function to split a string into words using a specified pattern.
const words = (str, pattern = /[^a-zA-Z-]+/) => {
// Split the input string using the specified pattern.
// Filter out any empty strings from the resulting array.
return str.split(pattern).filter(Boolean);
};
// Test the 'words' function with different input strings.
console.log(words('I love javaScript!!')); // Output: ['I', 'love', 'javaScript']
console.log(words('python, java, php')); // Output: ['python', 'java', 'php']
Output:
["I","love","javaScript"] ["python","java","php"]
Visual Presentation:
Flowchart:
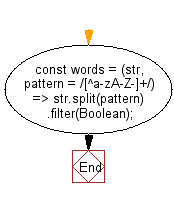
Live Demo:
See the Pen javascript-basic-exercise-103-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that splits a string into an array of words based on spaces and punctuation.
- Write a JavaScript function that trims and splits a sentence into words while filtering out empty strings.
- Write a JavaScript program that converts a paragraph into an array of words, accounting for multiple consecutive whitespace characters.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an array of elements, grouped based on the position in the original arrays.
Next: Write a JavaScript program to test a value, x, against a predicate function. If true, return fn(x). Else, return x.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.