JavaScript: Create an array of elements, grouped based on the position in the original arrays
JavaScript fundamental (ES6 Syntax): Exercise-102 with Solution
Group Array Elements by Position
Write a JavaScript program to create an array of elements, grouped based on the position in the original array.
- Use Math.max(), Function.prototype.apply() to get the longest array in the arguments.
- Create an array with that length as return value and use Array.from() with a mapping function to create an array of grouped elements.
- If lengths of the argument arrays vary, undefined is used where no value could be found.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'zip' function to zip arrays together.
const zip = (...arrays) => {
// Find the maximum length among the input arrays.
const maxLength = Math.max(...arrays.map(x => x.length));
// Use Array.from with a length equal to the maxLength to create the zipped array.
return Array.from({ length: maxLength }, (_, i) => {
// For each index 'i', map over the 'arrays' to extract the value at index 'i' for each array.
return arrays.map(arr => arr[i]);
});
};
// Test the 'zip' function with different arrays.
console.log(zip(['a', 'b'], [1, 2], [true, false]));
console.log(zip(['a'], [1, 2], [true, false]));
Output:
[["a",1,true],["b",2,false]] [["a",1,true],[null,2,false]]
Flowchart:
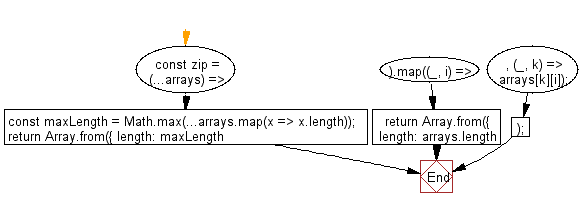
Live Demo:
See the Pen javascript-basic-exercise-102-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that groups elements of an array based on their index positions, returning an array of groups.
- Write a JavaScript function that splits an array into subarrays where each subarray contains elements from the same positions across multiple arrays.
- Write a JavaScript program that reorganizes an array into a two-dimensional array by grouping elements with the same index modulo a given number.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to return the object associating the properties to the values of an given array of valid property identifiers and an array of values.
Next: Write a JavaScript program to convert a given string into an array of words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.