JavaScript: Extract out the values at the specified indexes from a specified array
JavaScript fundamental (ES6 Syntax): Exercise-10 with Solution
Write a JavaScript program to extract values at specified indexes from a specified array.
- Use Array.prototype.filter() and Array.prototype.includes() to pull out the values that are not needed.
- Set Array.prototype.length to mutate the passed in an array by resetting its length to 0.
- Use Array.prototype.push() to re-populate it with only the pulled values.
- Use Array.prototype.push() to keep track of pulled values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called 'pull_at_Index' that takes two parameters: an array `arr` and an array of indices 'pullArr'.
const pull_at_Index = (arr, pullArr) => {
// Initialize an empty array to store removed elements.
let removed = [];
// Filter the array 'arr' based on the indices specified in 'pullArr'.
let pulled = arr
.map((v, i) => (pullArr.includes(i) ? removed.push(v) : v)) // Push the elements at specified indices to 'removed' array.
.filter((v, i) => !pullArr.includes(i)); // Filter out the elements at specified indices from 'arr'.
// Clear the original array 'arr' and replace it with the filtered elements.
arr.length = 0;
pulled.forEach(v => arr.push(v));
// Return the removed elements.
return removed;
};
// Test cases
let arra1 = ['a', 'b', 'c', 'd', 'e', 'f'];
console.log(pull_at_Index(arra1, [1, 3])); // Output: ['b', 'd']
let arra2 = [1, 2, 3, 4, 5, 6, 7];
console.log(pull_at_Index(arra2, [4])); // Output: [5]
Output:
["b","d"] [5]
Visual Presentation:
Flowchart:
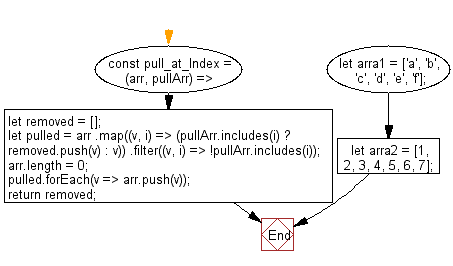
Live Demo:
See the Pen javascript-basic-exercise-1-10 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to combine the numbers of a given array into an array containing all combinations.
Next: Write a JavaScript program to generate a random hexadecimal color code.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics