JavaScript: Compare two objects to determine if the first one contains equivalent property values to the second one
JavaScript fundamental (ES6 Syntax): Exercise-1 with Solution
Compare Objects for Equivalent Properties
Write a JavaScript program to compare two objects to determine if the first contains equivalent property values to the second one.
- Use Object.keys() to get all the keys of the second object.
- Use Array.prototype.every(), Object.prototype.hasOwnProperty() and strict comparison to determine if all keys exist in the first object and have the same values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `matches` that takes two objects as arguments and checks if the first object contains all the key-value pairs of the second object.
const matches = (obj, source) =>
// Iterate over each key in the source object and check if it exists in the obj object and has the same value.
Object.keys(source).every(key => obj.hasOwnProperty(key) && obj[key] === source[key]);
// Test cases:
console.log(matches({ age: 25, hair: 'long', beard: true }, { hair: 'long', beard: true })); // true
console.log(matches({ hair: 'long', beard: true }, { age: 25, hair: 'long', beard: true })); // false
console.log(matches({ hair: 'long', beard: true }, { age: 26, hair: 'long', beard: true })); // false
Output:
true false false
Flowchart:
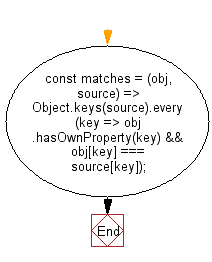
Live Demo:
See the Pen javascript-basic-exercise-1-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that deeply compares two objects, including nested properties, and returns true if they are equivalent.
- Write a JavaScript function that checks if all property values in one object are contained in another, ignoring extra properties.
- Write a JavaScript program that compares two objects for equivalent property values but ignores properties with undefined values.
Improve this sample solution and post your code through Disqus
Previous: JavaScript fundamental Exercises
Next: Write a JavaScript program to copy a string to the clipboard.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics