JavaScript Program: Handling URIError with Try-Catch block for invalid URI decoding
JavaScript Error Handling: Exercise-9 with Solution
Write a JavaScript program that uses a try-catch block to catch and handle a `URIError` when decoding an invalid URI.
Sample Solution:
JavaScript Code:
// Define a function named decode_URI_String that takes a parameter uriString
function decode_URI_String(uriString) {
// Attempt to decode the URI string
try {
// Decode the URI string
const decodedURI = decodeURI(uriString);
// Log the decoded URI to the console
console.log('Decoded URI:', decodedURI);
} catch (error) {
// If an error occurs during execution
// Check if the error is a URIError
if (error instanceof URIError) {
// If the error is a URIError, log the error message with 'URIError' prefix
console.log('URIError:', error.message);
} else {
// If the error is not a URIError, log the error message with 'Error' prefix
console.log('Error:', error.message);
}
}
}
// Example:
// Call the decode_URI_String function with a valid URI string
decode_URI_String('https://example.com/'); // Valid URI
// Call the decode_URI_String function with an invalid URI string containing special characters
decode_URI_String('https://example.com/%%invalidURI'); // Invalid URI
Output:
"Decoded URI:" "https://example.com/" "URIError:" "URI malformed"
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The "decodeURIString()" function attempts to decode the provided URIString using the decodeURI function within a try block.
- If the URI is valid, the decoding process will succeed, and the decoded URI will be logged to the console.
- However, if the URI is invalid, a URIError will be thrown. We catch this error in the catch block and check if the error is an instance of URIError using the instanceof operator.
- If it is a URIError, we log a message with the prefix 'URIError:' followed by the error message. Otherwise, for other types of error, we log a message with the prefix 'Error:' followed by the error message.
Next we call the “decodeURIString()” function twice. The first call with a valid URI 'https://example.com/' will decode successfully and print the decoded URI to the console.
The second call with an invalid URI 'https://example.com/%%invalidURI' will throw a URIError due to invalid characters. The catch block will handle this error and print a message indicating the URIError.
Flowchart:
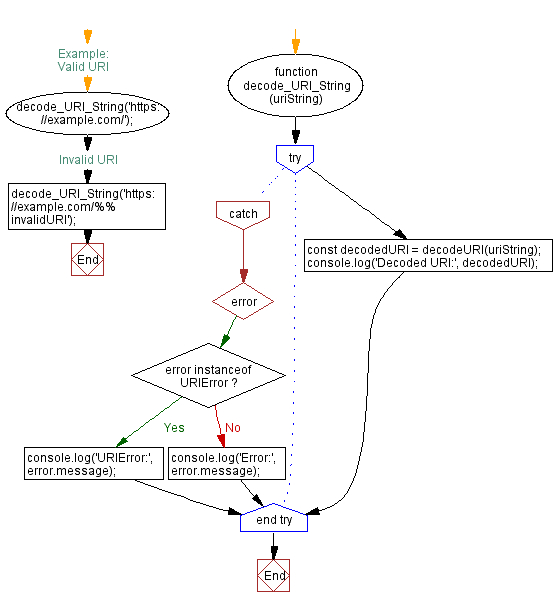
Live Demo:
See the Pen javascript-error-handling-exercise-9 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Handling different types of errors with multiple catch blocks.
Error Handling Exercises Next: Error handling and cleanup with the try-catch-finally statement.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/error-handling/javascript-error-handling-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics