JavaScript Function: Custom Error on the second number zero
JavaScript Error Handling: Exercise-3 with Solution
Throw Error for Division by Zero
Write a JavaScript function that accepts two numbers as parameters and throws a custom 'Error' if the second number is zero.
Sample Solution:
JavaScript Code:
// Define a function named divide_Numbers that takes two parameters: n1 and n2
function divide_Numbers(n1, n2) {
// Check if n2 is equal to 0
if (n2 === 0) {
// If n2 is 0, throw an error indicating division by zero is not allowed
throw new Error('Error: Division by zero is not allowed.');
}
// If n2 is not 0, return the result of dividing n1 by n2
return n1 / n2;
}
// Call the divide_Numbers function with arguments 8 and 3, then log the result to the console
console.log(divide_Numbers(8, 3));
// Call the divide_Numbers function with arguments 8 and 0, which will throw an error, and handle it
console.log(divide_Numbers(8, 0));
Output:
2.6666666666666665 "error" "Error: Error: Division by zero is not allowed.
Note: Executed on JS Bin
Explanation:
In the above function "divide_Numbers()", we first check if n2 is equal to zero. If it is, we throw a custom Error with the message 'Error: Division by zero is not allowed.' This prevents the function from performing a division by zero operation, which would result in an error.
If n2 is not zero, the function continues and calculates the division of n1 by n2 using the division operator (/). The result is then returned.
Flowchart:
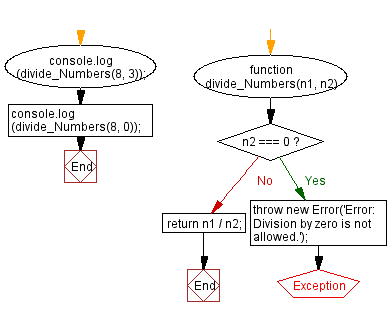
Live Demo:
See the Pen javascript-error-handling-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that performs division on two numbers and throws a custom Error if the divisor is zero or nearly zero (using an epsilon check).
- Write a JavaScript function that sequentially divides a list of numbers and throws an Error immediately upon encountering a division by zero.
- Write a JavaScript function that uses recursion to perform division and throws an Error if any recursive call attempts to divide by zero.
- Write a JavaScript function that processes user input for division and validates the divisor, throwing a custom Error if it is zero.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Handling TypeError with Try-Catch block.
Error Handling Exercises Next: Custom Error on a negative number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics