JavaScript Program: Catching and handling EvalError with the try-catch Block
JavaScript Error Handling: Exercise-11 with Solution
Handle EvalError
Write a JavaScript program that uses a try-catch block to catch and handle an 'EvalError' when evaluating an invalid expression.
Sample Solution:
JavaScript Code:
// Define a function named evaluate_Expression that takes a string expression (exp) as input
function evaluate_Expression(exp) {
// Try block to evaluate the expression and handle potential errors
try {
// Evaluate the expression using the eval function
const result = eval(exp);
// Log the result to the console
console.log('Result:', result);
} catch (error) {
// Catch block to handle errors
// Check if the error is an instance of EvalError
if (error instanceof EvalError) {
// If the error is an EvalError, log the error message to the console
console.log('EvalError:', error.message);
} else {
// If the error is not an EvalError, log the error message to the console
console.log('Error:', error.message);
}
}
}
// Example:
// Call the evaluate_Expression function with a valid expression
evaluate_Expression('30 + 30'); // Valid expression
// Call the evaluate_Expression function with an invalid expression
evaluate_Expression('3 +'); // Invalid expression
Output:
"Result:" 60 "Error:" "Unexpected end of input"
Note: Executed on JS Bin
Explanation:
In the above exercise -
The "evaluate_Expression()" function takes an expression as a parameter and evaluates it using the "eval()" function.
- Inside the try block, the code uses eval(exp) to evaluate the provided expression. The result is stored in the result variable.
- If the expression is valid and can be successfully evaluated, the result is logged to the console using console.log('Result:', result).
- If an error occurs during evaluation, the code jumps to the catch block.
- Inside the catch block, the error object is caught in the error parameter.
- The code checks if the error is an instance of EvalError using the instanceof operator.
- If it is, the error message is logged to the console as 'EvalError: <error message>'.
- If it's a different type of error, the error message is logged as 'Error: <error message>'.
Finally evaluate_Expression('30 + 30') returns a valid expression, '30 + 30', which results in 60 being logged to the console.
Next evaluate_Expression('3 +'), returns an invalid expression missing an operand. This causes an EvalError to be thrown. The error message, 'Unexpected end of input', is logged to the console.
Flowchart:
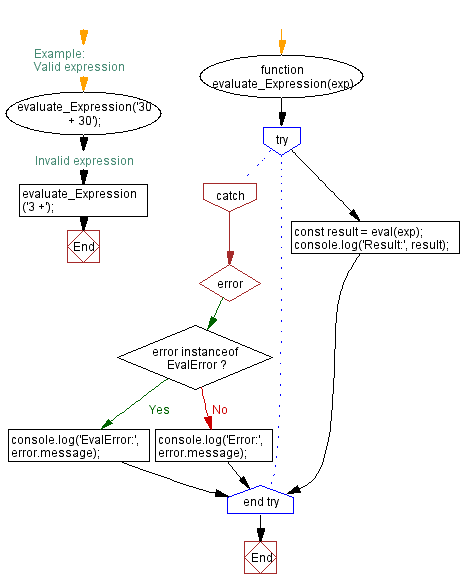
Live Demo:
See the Pen javascript-error-handling-exercise-11 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that uses eval on an improperly formatted expression to trigger and catch an EvalError.
- Write a JavaScript function that deliberately misuses eval in strict mode and handles the EvalError using try-catch.
- Write a JavaScript function that dynamically constructs code strings for eval and catches any EvalError arising from malformed syntax.
- Write a JavaScript function that simulates dynamic code execution via eval and gracefully manages potential EvalErrors without crashing.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Error handling and cleanup with the try-catch-finally statement.
Error Handling Exercises Next: Catching and handling ReferenceError with the try-catch Block.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics