JavaScript - Binary logarithm using bitwise operators
JavaScript Bit Manipulation: Exercise-8 with Solution
Binary Logarithm (log2n)
Write a JavaScript program to calculate the binary logarithm (log2n) using bitwise operators.
From handwiki.org -
Binary logarithm:
In mathematics, the binary logarithm (log2n) is the power to which the number 2 must be raised to obtain the value n. That is, for any real number x,
For example, the binary logarithm of 1 is 0, the binary logarithm of 2 is 1, the binary logarithm of 4 is 2, and the binary logarithm of 32 is 5.
The binary logarithm is the logarithm to the base 2 and is the inverse function of the power of two function. As well as log2, an alternative notation for the binary logarithm is lb (the notation preferred by ISO 31-11 and ISO 80000-2).
Test Data:
(1) -> 0
(2) -> 1
(4) -> 2
(32) -> 5
Sample Solution:
JavaScript Code:
// Define a function to calculate the logarithm base 2 of a number
const log_Two = (n) => {
// Check if the input is not a number
if (typeof n!= "number") {
return 'It must be number!' // Return an error message
}
let result = 0; // Initialize the result variable to 0
// Loop to find the logarithm base 2 of the number
while (n >> 1) {
n >>= 1; // Right shift the number by 1 bit
result++; // Increment the result counter
}
return result; // Return the logarithm base 2 of the number
}
console.log(log_Two(1)) // Test the function with the number 1
console.log(log_Two(2)) // Test the function with the number 2
console.log(log_Two(4)) // Test the function with the number 4
console.log(log_Two(32)) // Test the function with the number 32
Output:
0 1 2 5
Flowchart:
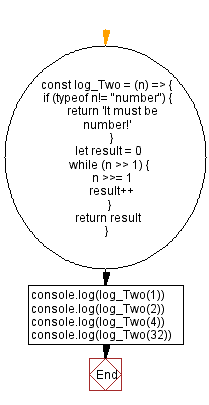
Live Demo:
See the Pen javascript-bit-manipulation-exercise-8 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the binary logarithm of a number using bitwise operations to count the number of shifts.
- Write a JavaScript function that uses recursion to compute log base 2 of a number without Math.log2.
- Write a JavaScript function that validates the input is a power of two and returns the exponent if true, or an approximation otherwise.
- Write a JavaScript function that compares the result of your bitwise log2 implementation with Math.log2 for accuracy.
Improve this sample solution and post your code through Disqus.
Previous: Swap two bits at given position in an integer.
Next: Turn off the kth bit of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.