JavaScript - Next power of two of a given number
JavaScript Bit Manipulation: Exercise-4 with Solution
Next Power of Two
Write a JavaScript program to find the next power of two of a given number.
A power of two is a number of the form 2n where n is an integer, that is, the result of exponentiation with number two as the base and integer n as the exponent.
The first ten powers of 2 for non-negative values of n are: 1, 2, 4, 8, 16, 32, 64, 128, 256, 512, ...
Test Data:
(1) -> 1
(4) -> 4
(9) -> 16
("15") -> "It must be number!"
Sample Solution:
JavaScript Code:
Output:
1 4 16 It must be number!
Flowchart:
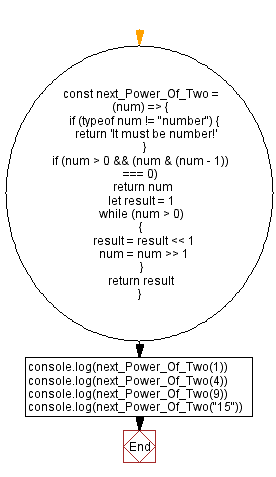
Live Demo:
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the next power of two greater than or equal to a given number using bit manipulation.
- Write a JavaScript function that iteratively doubles a value until it is greater than or equal to the input number.
- Write a JavaScript function that validates the input and returns an error if it is not a number.
- Write a JavaScript function that uses logarithms and Math.ceil to compute the next power of two.
Improve this sample solution and post your code through Disqus.
Previous: Number of 0 bits in a binary representation.
Next: Odd or even number using bit manipulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics