JavaScript - Number of 0 bits in a binary representation
JavaScript Bit Manipulation: Exercise-3 with Solution
Count Zero Bits
Write a JavaScript program to count 0 bits in the binary representation of a given integer.
Test Data:
(45) -> 2
(17) -> 3
(15) -> "Parameter value is not an Integer!"
Sample Solution:
JavaScript Code:
// Define a function to count the number of set bits (1s) in the binary representation of a given integer
function Binary_Count_SetBits(a)
{
// Check whether the input is an integer
if (!Number.isInteger(a))
{
return('Parameter value is not an Integer!') // Return an error message if the input is not an integer
}
// Convert the integer to its binary representation, split it by '0', and count the number of substrings
return a.toString(2).split('0').length - 1
}
console.log(Binary_Count_SetBits(45)) // Test the function with the integer 45
console.log(Binary_Count_SetBits(17)) // Test the function with the integer 17
console.log(Binary_Count_SetBits("15")) // Test the function with the string "15"
Output:
2 3 Parameter value is not an Integer!
Flowchart:
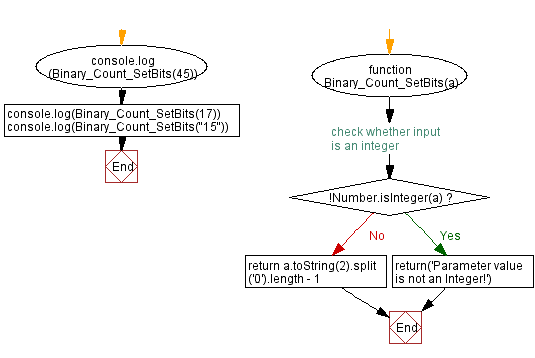
Live Demo:
See the Pen javascript-bit-manipulation-exercise-2 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a given integer to its binary representation and counts the number of 0 bits.
- Write a JavaScript function that iterates over each bit of an integer using bit shifting to count zeros.
- Write a JavaScript function that validates if the input is a non-negative integer before performing the count.
- Write a JavaScript function that uses recursion to count zero bits in the binary representation of a number.
Improve this sample solution and post your code through Disqus.
Previous: Swap two variables using bit manipulation.
Next: Next power of two of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.