JavaScript - Find the non-repeated element from an array
JavaScript Bit Manipulation: Exercise-14 with Solution
Non-Repeated Element
In an array every element appears twice except for one. Write a JavaScript program to find the non-repeated element in an array using bit manipulation.
Test Data:
([1]) -> 1
([1, 2, 3]) -> 0 [All elements are non- repeated]
[1, 2, 8, 3, 1, 2, 3, 8, 6, 6, 7] -> 7
Sample Solution:
JavaScript Code:
// Define a function to find the non-repeated number in an array of integers
const non_repeated_num = (nums) => {
let r = 0; // Initialize a variable to store the result
// Iterate through the array elements
for(let i = 0; i <= nums.length; i++) {
r = r ^ nums[i]; // Use bitwise XOR operation to find the non-repeated number
}
return r; // Return the non-repeated number
}
// Define an array of integers
nums = [1, 2, 8, 3, 1, 2, 3, 8, 6, 6, 7];
// Call the non_repeated_num function to find the non-repeated number in the array
console.log(non_repeated_num(nums));
Output:
7
Flowchart:
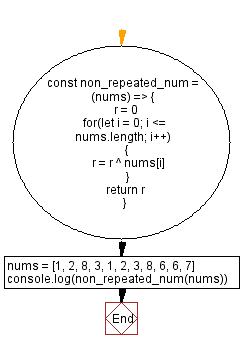
Live Demo:
See the Pen javascript-bit-manipulation-exercise-14 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
Improve this sample solution and post your code through Disqus.
Previous: Parity of a given number.
Next: Maximum, minimum of two integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics